API Blueprint tutorial
This tutorial will take you through the basics of the API Blueprint language. We’re going to build an API Blueprint step by step.
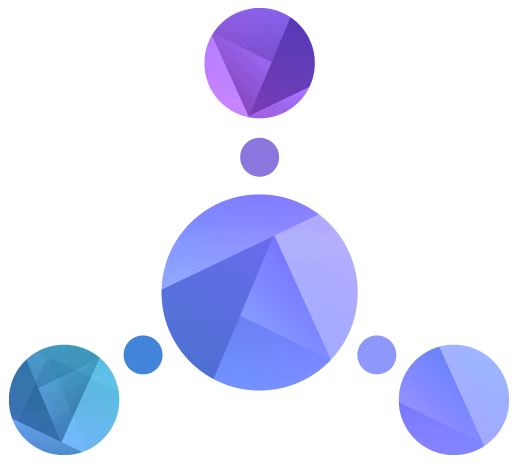
API Blueprint
API Blueprint is open sourced format for describing APIs.
This is how API Blueprint looks like:
FORMAT: 1A
# Polls
Polls is a simple API allowing consumers to view polls and vote in them.
## Questions Collection [/questions]
### List All Questions [GET]
+ Response 200 (application/json)
{
"question": "Favourite programming language?",
"choices": [
{
"choice": "Swift",
"votes": 2048
}, {
"choice": "Python",
"votes": 1024
}
]
}
Recommended file extension for API Blueprint is .apib
Writing API Blueprint
Choosing editor
If you are new to API Blueprint, best choice is to use Apiary Editor on Apiary.io, because of its built-in helpers and instant preview.
Metadata, API Name & Description
The first step for creating a Blueprint is to specify the API Name and metadata:
FORMAT: 1A
# Polls
Polls is a simple API allowing consumers to view polls and vote in them.
The Blueprint starts with a metadata section. The FORMAT
keyword is required and denotes that document is API Blueprint.
You can also add HOST
, which should have value of your production API URI. This will allow you to test and debug production calls from Apiary.
First level heading # Polls
will become API name.
Resource
API consists of resources specified by their URIs. In this example we have a resource called Question Collection
, which allows you to view a list of questions. The heading specifies its URI inside of square brackets [/questions]
.
## Question Collection [/questions]
Actions
You should specify each action you may make on a resource. An action is specified with a sub-heading with the name of the action followed by the HTTP method.
## Question Collection [/questions]
### List All Questions [GET]
An action should include at least one response from the server
which must include a status code and may contain a body.
A response is defined as a list item within an action.
Lists are created by preceding list items with either a +, * or -.
This action returns a 200 status code along with a JSON body.
+ Response 200 (application/json)
{
"question": "Favourite programming language?",
"published_at": "2014-11-11T08:40:51.620Z",
"url": "/questions/1",
"choices": [
{
"choice": "Swift",
"url": "/questions/1/choices/1",
"votes": 2048
}, {
"choice": "Python",
"url": "/questions/1/choices/2",
"votes": 1024
}, {
"choice": "Objective-C",
"url": "/questions/1/choices/3",
"votes": 512
}, {
"choice": "Ruby",
"url": "/questions/1/choices/4",
"votes": 256
}
]
}
Specifying the media type after the response status code generates a Content-Type
HTTP header. You do not have to specify it manually in Headers
section.
The polls resource has a second action which allows you to create a new question. This action shows the structure you should send to the server to perform this action.
### Create a New Question [POST]
You may create your own question using this action.
This action takes a JSON payload as part of the request.
Response then return specific header and body.
+ Request (application/json)
{
"question": "Favourite programming language?",
"choices": [
"Swift",
"Python",
"Objective-C",
"Ruby"
]
}
+ Response 201 (application/json)
+ Headers
Location: /questions/1
+ Body
{
"question": "Favourite programming language?",
"published_at": "2014-11-11T08:40:51.620Z",
"url": "/questions/1",
"choices": [
{
"choice": "Swift",
"url": "/questions/1/choices/1",
"votes": 0
}, {
"choice": "Python",
"url": "/questions/1/choices/2",
"votes": 0
}, {
"choice": "Objective-C",
"url": "/questions/1/choices/3",
"votes": 0
}, {
"choice": "Ruby",
"url": "/questions/1/choices/4",
"votes": 0
}
]
}
Single resource with parameters
The next resource is Question
, which represents a single question.
## Question [/questions/{question_id}]
+ Parameters
+ question_id (number) - ID of the Question in the form of an integer
URI Template
The URI for the Question
resource uses a variable component {question_id}
, expressed by URI Template.
URI Parameters
URI parameters + Parameters
should describe the URI using a list of Parameters.
The question_id
variable of the URI template is a parameter for every action on this resource. Here it’s defined as type number
, followed by its description.
With action:
## Question [/questions/{question_id}]
+ Parameters
+ question_id (number) - ID of the Question in the form of an integer
### View a Questions Detail [GET]
+ Response 200 (application/json)
{
"question": "Favourite programming language?",
"published_at": "2014-11-11T08:40:51.620Z",
"url": "/questions/1",
"choices": [
{
"choice": "Swift",
"url": "/questions/1/choices/1",
"votes": 2048
}, {
"choice": "Python",
"url": "/questions/1/choices/2",
"votes": 1024
}, {
"choice": "Objective-C",
"url": "/questions/1/choices/3",
"votes": 512
}, {
"choice": "Ruby",
"url": "/questions/1/choices/4",
"votes": 256
}
]
}
Once you add this Blueprint into Apiary, you will instantly get a Mock server, Traffic inspector, Interactive console and much more for your API.
Advanced usage
Resource Groups
You can group related resources by using heading with the Group
keyword.
FORMAT: 1A
# Polls
Polls is a simple API allowing consumers to view polls and vote in them.
# Group Questions
Resources related to questions in the API.
Response Without a Body
This resource has a delete action. The server will return a 204 response without a body.
## Question [/questions/{question_id}]
+ Parameters
+ question_id (number) - ID of the Question in the form of an integer
### Delete [DELETE]
+ Response 204
Multiple responses
You can specify different responses and even different requests on each action. Multiple responses are supported within Mock server. It is especially suitable for handling multiple types of Content-Type
header.
### Retrieve Message [GET]
+ Request
+ Headers
Authorization: Basic ABCDEF
+ Response 200 (application/json)
{
"message":"Hello World!"
}
+ Request (application/json)
+ Body
{
"grant_type": "client_credentials"
}
+ Response 401 (text/plain)
There is no such a message for you, dear guest.
+ Response 410 (text/plain)
The message you are searching for does not exist anymore.
If you are responding differently based on parameters/query string arguments, you should use URI templates.